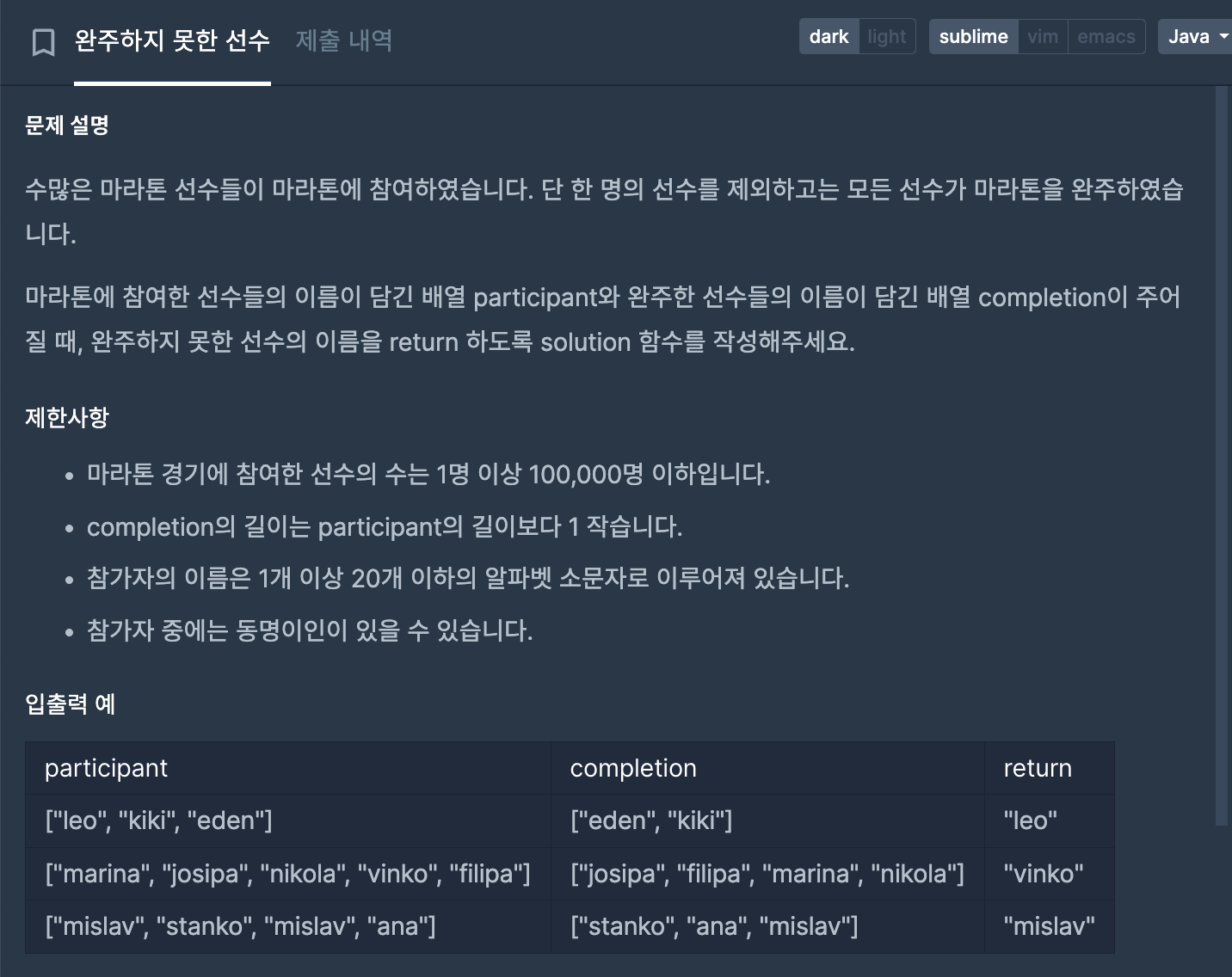
https://school.programmers.co.kr/learn/courses/30/lessons/42576?language=java
프로그래머스
SW개발자를 위한 평가, 교육, 채용까지 Total Solution을 제공하는 개발자 성장을 위한 베이스캠프
programmers.co.kr
처음에는 HashMap으로 풀었다.
정확성 테스트는 모두 통과했지만, 효율성 테스트는 전체 5개 중 하나밖에 통과하지 못했다.
HashMap 사용 (효율성 테스트 통과 X)
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
String answer = "";
HashMap<String, Integer> pMap = new HashMap<>();
HashMap<String, Integer> cMap = new HashMap<>();
for (String part : participant) {
pMap.put(part, pMap.getOrDefault(part, 0) + 1);
}
for (String comp : completion) {
cMap.put(comp, cMap.getOrDefault(comp, 0) + 1);
}
for (String part : participant) {
if (pMap.getOrDefault(part, 0) != cMap.getOrDefault(part, 0)) {
answer = part;
break;
}
}
return answer;
}
}
HashMap 사용 (효율성 테스트 통과 O)
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
String answer = "";
HashMap<String, Integer> map = new HashMap<>();
for (String part : participant) {
map.put(part, map.getOrDefault(part, 0) + 1);
}
for (String comp : completion) {
map.put(comp, map.getOrDefault(comp, 0) - 1);
}
for (String part : participant) {
if (map.getOrDefault(part, 0) != 0) {
answer = part;
break;
}
}
return answer;
}
}
두 개의 map을 사용하지 않고,
완주한 선수들의 경우 기존 값에서 1씩 빼주는 방법을 사용하니
효율성 테스트를 통과했다.
정렬 사용
구글링 해서 알게 된 방법이다.
두 배열을 모두 정렬한 뒤,
i번째 값이 같지 않다면 participant[i]를 리턴해주면 된다.
완주자 명단에 없는 값이 participant의 가장 마지막 값일 가능성도 있으므로,
마지막에 participant[participant.length - 1] 리턴도 추가해줬다.
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
Arrays.sort(participant);
Arrays.sort(completion);
for (int i = 0; i < completion.length; i++) {
if (!completion[i].equals(participant[i])) {
return participant[i];
}
};
return participant[participant.length - 1];
}
}
'Languages > Java' 카테고리의 다른 글
[BOJ/Java] #11279 최대 힙 - PriorityQueue (0) | 2025.03.24 |
---|---|
[프로그래머스 고득점 Kit/Java] 폰켓몬 (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 11일차 #5430 AC (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 10일차 #1158 요세푸스 문제 (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 11일차 #1966 프린터 큐 (0) | 2025.03.23 |
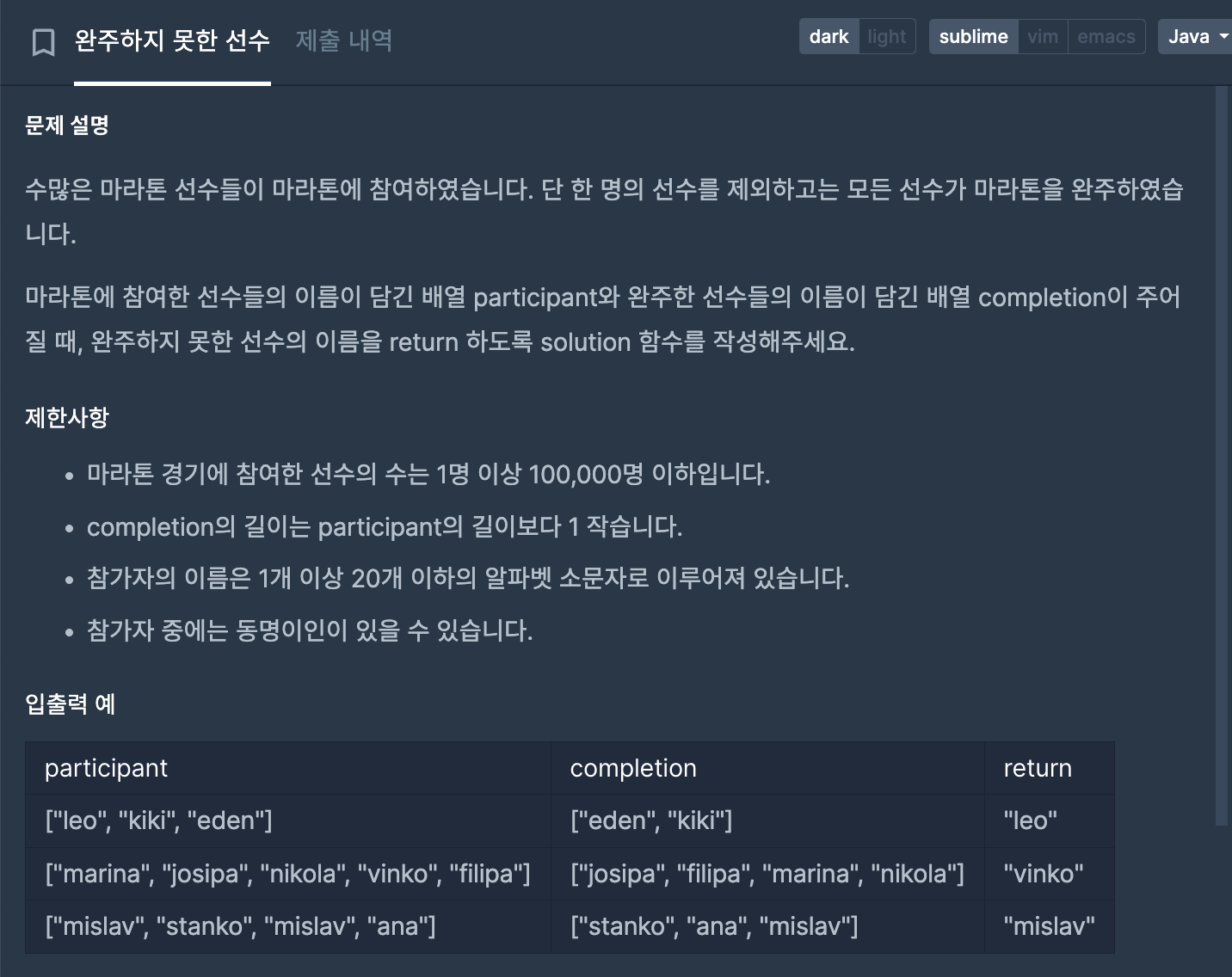
https://school.programmers.co.kr/learn/courses/30/lessons/42576?language=java
프로그래머스
SW개발자를 위한 평가, 교육, 채용까지 Total Solution을 제공하는 개발자 성장을 위한 베이스캠프
programmers.co.kr
처음에는 HashMap으로 풀었다.
정확성 테스트는 모두 통과했지만, 효율성 테스트는 전체 5개 중 하나밖에 통과하지 못했다.
HashMap 사용 (효율성 테스트 통과 X)
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
String answer = "";
HashMap<String, Integer> pMap = new HashMap<>();
HashMap<String, Integer> cMap = new HashMap<>();
for (String part : participant) {
pMap.put(part, pMap.getOrDefault(part, 0) + 1);
}
for (String comp : completion) {
cMap.put(comp, cMap.getOrDefault(comp, 0) + 1);
}
for (String part : participant) {
if (pMap.getOrDefault(part, 0) != cMap.getOrDefault(part, 0)) {
answer = part;
break;
}
}
return answer;
}
}
HashMap 사용 (효율성 테스트 통과 O)
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
String answer = "";
HashMap<String, Integer> map = new HashMap<>();
for (String part : participant) {
map.put(part, map.getOrDefault(part, 0) + 1);
}
for (String comp : completion) {
map.put(comp, map.getOrDefault(comp, 0) - 1);
}
for (String part : participant) {
if (map.getOrDefault(part, 0) != 0) {
answer = part;
break;
}
}
return answer;
}
}
두 개의 map을 사용하지 않고,
완주한 선수들의 경우 기존 값에서 1씩 빼주는 방법을 사용하니
효율성 테스트를 통과했다.
정렬 사용
구글링 해서 알게 된 방법이다.
두 배열을 모두 정렬한 뒤,
i번째 값이 같지 않다면 participant[i]를 리턴해주면 된다.
완주자 명단에 없는 값이 participant의 가장 마지막 값일 가능성도 있으므로,
마지막에 participant[participant.length - 1] 리턴도 추가해줬다.
import java.util.*;
import java.io.*;
class Solution {
public String solution(String[] participant, String[] completion) {
Arrays.sort(participant);
Arrays.sort(completion);
for (int i = 0; i < completion.length; i++) {
if (!completion[i].equals(participant[i])) {
return participant[i];
}
};
return participant[participant.length - 1];
}
}
'Languages > Java' 카테고리의 다른 글
[BOJ/Java] #11279 최대 힙 - PriorityQueue (0) | 2025.03.24 |
---|---|
[프로그래머스 고득점 Kit/Java] 폰켓몬 (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 11일차 #5430 AC (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 10일차 #1158 요세푸스 문제 (0) | 2025.03.23 |
[BOJ 길라잡이/Java] 11일차 #1966 프린터 큐 (0) | 2025.03.23 |